PHP WordPress
WordPress - Adding Custom Post Types & Taxonomies
562 days agoThe code below shows you how to add Custom Post Types and their Taxonomies.
<?php
add_action('init', 'hoog_register_post_types');
function hoog_register_post_types()
{
$supports = [
'title', // post title
'editor', // post content
'author', // post author
'thumbnail', // featured images
'excerpt', // post excerpt
'revisions', // post revisions
'post-formats', // post formats
];
$labels = [
'name' => _x('vacancies', 'plural'),
'singular_name' => _x('vacancy', 'singular'),
'menu_name' => _x('vacancies', 'admin menu'),
'name_admin_bar' => _x('vacancy', 'admin bar'),
'add_new' => _x('Add New', 'add new'),
'add_new_item' => __('Add Vacancy'),
'new_item' => __('New Vacancy'),
'edit_item' => __('Edit vacancy'),
'view_item' => __('View vacancy'),
'all_items' => __('All vacancies'),
'search_items' => __('Search vacancies'),
'not_found' => __('No vacancies found.'),
];
$args = [
'supports' => $supports,
'labels' => $labels,
'public' => true,
'query_var' => true,
'rewrite' => array('slug' => 'vacancies'),
'has_archive' => true,
'hierarchical' => false,
];
register_post_type('vacancies', $args);
}
add_action( 'init', 'hoog_register_taxonomies', 0 );
function hoog_register_taxonomies()
{
$labels = [
'name' => _x('sectors', 'taxonomy general name'),
'singular_name' => _x('Sector', 'taxonomy singular name'),
'search_items' => __('Search Sectors'),
'all_items' => __('All Sectors'),
'parent_item' => __('Parent Sector'),
'parent_item_colon' => __('Parent Sector:'),
'edit_item' => __('Edit Sector'),
'update_item' => __('Update Sector'),
'add_new_item' => __('Add New Sector'),
'new_item_name' => __('New Sector Name'),
'menu_name' => __('Sectors'),
];
// Add new "Locations" taxonomy to Posts
register_taxonomy('sector', 'post', [
// Hierarchical taxonomy (like categories)
'hierarchical' => true,
// This array of options controls the labels displayed in the WordPress Admin UI
'labels' => $labels,
// Control the slugs used for this taxonomy
'rewrite' = [
'slug' = 'sectors', // This controls the base slug that will display before each term
'with_front' => true,
'hierarchical' => true
],
]);
}
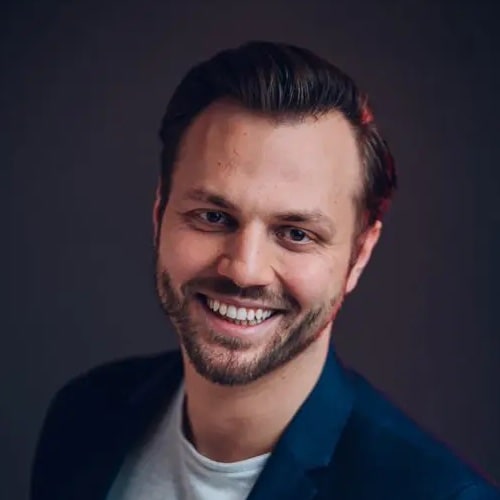